How to Write Clean and Maintainable Code: Best Practices for Developers
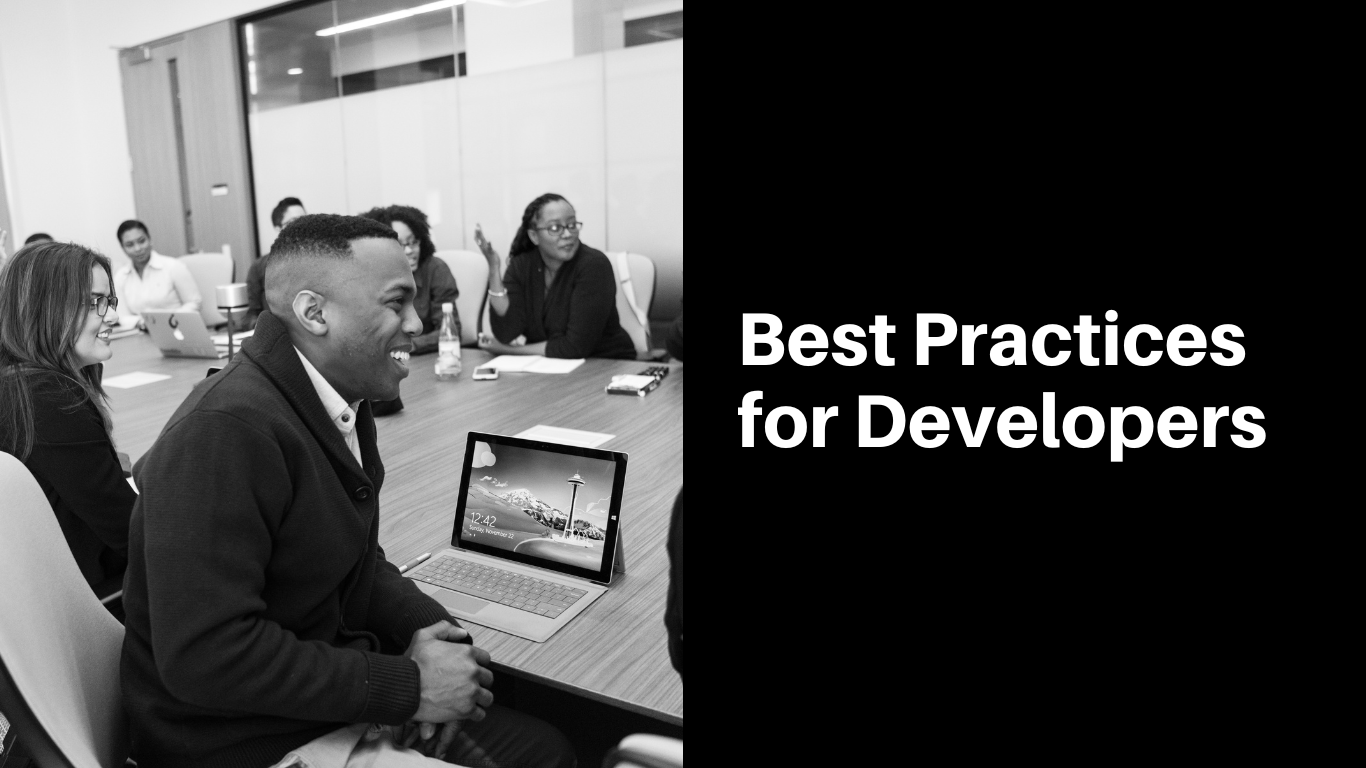
Writing clean and maintainable code is a critical skill for developers. Clean code is easier to read, debug, and extend, making it more efficient for teams to work collaboratively. Here are some best practices to ensure your code stays clean and maintainable:
1. Follow Consistent Naming Conventions
- Use meaningful and descriptive names for variables, functions, and classes.
- Stick to a naming convention, such as camelCase for variables (
myVariable
) and PascalCase for classes (MyClass
). - Avoid single-letter or ambiguous names (e.g.,
x
,data
) unless they are well understood in context.
Example:
// Bad
let x = 10;
function d() {
return x * x;
}
// Good
let sideLength = 10;
function calculateArea() {
return sideLength * sideLength;
}
2. Write Modular Code
- Break your code into smaller, reusable functions or modules.
- Follow the Single Responsibility Principle: each function or module should handle only one task.
Example:
# Bad: One function doing too much
def process_data(data):
cleaned_data = clean(data)
analyzed_data = analyze(cleaned_data)
save_to_db(analyzed_data)
# Good: Modular functions
def clean(data):
# Clean the data
pass
def analyze(data):
# Analyze the data
pass
def save_to_db(data):
# Save data to the database
pass
def process_data(data):
cleaned_data = clean(data)
analyzed_data = analyze(cleaned_data)
save_to_db(analyzed_data)
3. Comment and Document Your Code
- Write comments to explain why certain decisions were made, not just what the code does.
- Use tools like JSDoc, Sphinx, or Markdown files for documenting larger projects.
Example:
// Calculate the area of a rectangle given width and height
function calculateRectangleArea(width, height) {
return width * height;
}
4. Adopt a Consistent Code Style
- Use a linter (e.g., ESLint, Pylint) and formatter (e.g., Prettier, Black) to enforce style guidelines.
- Agree on a coding standard within your team, such as the Google Style Guide or Airbnb JavaScript Style Guide.
Example:
{
"rules": {
"indent": ["error", 2],
"quotes": ["error", "double"],
"semi": ["error", "always"]
}
}
5. Use Version Control Effectively
- Commit frequently with descriptive messages (e.g., “Add validation for user input”).
- Use feature branches to isolate work on specific tasks or features.
Example Commit Message:
feat: Add user authentication module
- Implement login and logout functionality
- Add token-based authentication
- Write unit tests for authentication module
6. Write Tests
- Write unit, integration, and end-to-end tests to ensure your code works as expected.
- Use testing frameworks like Jest (JavaScript), PyTest (Python), or JUnit (Java).
Example:
# Test for a function that calculates the square of a number
def test_square():
assert square(3) == 9
assert square(-4) == 16
assert square(0) == 0
7. Avoid Code Duplication
- DRY (Don’t Repeat Yourself): Extract reusable code into functions or modules.
Example:
// Bad: Duplicated logic
int calculateCircleArea(int radius) {
return 3.14 * radius * radius;
}
int calculateSphereVolume(int radius) {
return (4 / 3) * 3.14 * radius * radius * radius;
}
// Good: Shared constant and reusable function
final double PI = 3.14;
int calculateCircleArea(int radius) {
return PI * radius * radius;
}
int calculateSphereVolume(int radius) {
return (4 / 3) * PI * Math.pow(radius, 3);
}
8. Handle Errors Gracefully
- Use proper error handling to avoid unexpected crashes.
- Provide meaningful error messages for debugging.
Example:
// Bad: Silent failure
function fetchData() {
try {
// Fetch data
} catch (error) {
// Do nothing
}
}
// Good: Log and handle the error
function fetchData() {
try {
// Fetch data
} catch (error) {
console.error('Error fetching data:', error);
}
}
9. Refactor Regularly
- Revisit your code to improve readability, performance, or structure.
- Remove unused code and dependencies.
10. Stay Updated with Best Practices
- Follow industry blogs, podcasts, and communities to learn about new tools and techniques.
- Regularly review your team’s coding standards to incorporate improvements.
Conclusion
Clean and maintainable code is not just about writing code that works; it’s about writing code that other developers can understand and build upon. By following these best practices, you’ll create a solid foundation for long-term project success and effective team collaboration.