How to Build a Portfolio That Stands Out in the Web Dev Job Market
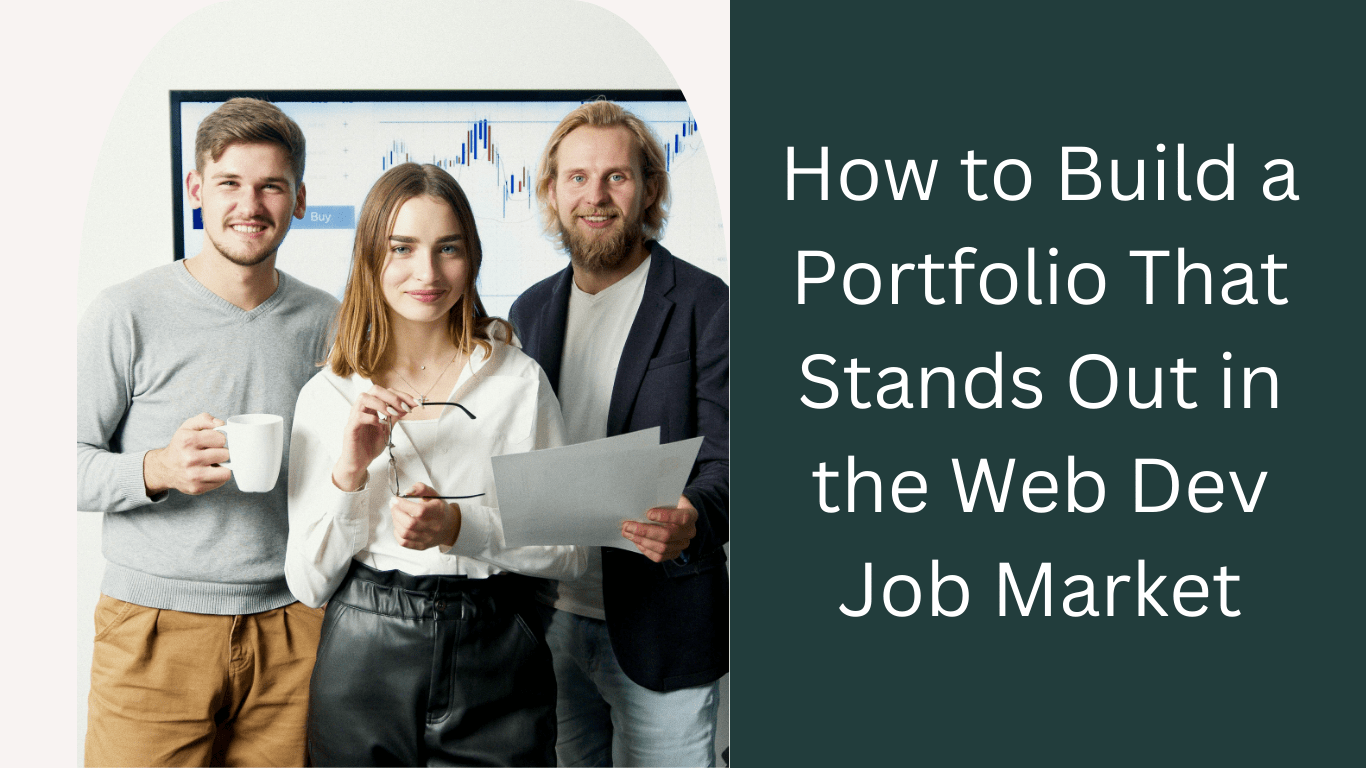
How to Build a Portfolio That Stands Out in the Web Dev Job Market
Web development requires not only technical skills but also a deep understanding of security practices to protect applications from potential threats. Here are some essential security practices every web developer should follow:
1. Crafting an Outstanding Portfolio
- Showcase Real Projects: Include live links to projects that demonstrate your skills in front-end, back-end, and full-stack development.
- Focus on Diversity: Highlight a variety of projects (e.g., e-commerce, personal blogs, dashboards) to show versatility.
- Include Source Code: Provide links to GitHub repositories for transparency and to showcase clean, maintainable code.
2. Highlight Your Best Work
- Select Quality Over Quantity: Focus on 3-5 standout projects rather than overwhelming with many examples.
- Tailor to the Audience: Highlight projects relevant to the jobs you’re applying for.
- Use Case Studies: Explain your thought process, challenges faced, and solutions implemented in each project.
3. Ensure Professional Presentation
- Clean and Responsive Design: Your portfolio itself should reflect your web design skills.
- Easy Navigation: Ensure that hiring managers can quickly find the information they need.
- Include a Personal Brand: Use a consistent color scheme, logo, and tone that reflect your personality.
4. Demonstrate Modern Practices
- Use modern frameworks (e.g., React, Vue, Angular) and technologies.
- Incorporate performance optimization techniques like lazy loading and responsive images.
- Show examples of API integration, authentication, or real-time features.
5. Add a Blog Section
- Write about web development topics, challenges, or tutorials.
- Use this space to demonstrate your problem-solving skills and passion for the field.
- Share insights on industry trends to position yourself as a thought leader.
6. Include Testimonials and Feedback
- Add client or colleague testimonials to boost credibility.
- If possible, include metrics (e.g., “Improved site performance by 30%”) to quantify your impact.
7. Optimize for Visibility
- SEO Optimization: Use meta tags, descriptive project titles, and alt text for images.
- Mobile-Friendly Design: Ensure your portfolio works seamlessly on all devices.
- Custom Domain: Invest in a custom domain name (e.g., yourname.dev) for a professional touch.
8. Keep It Updated
- Regularly update your portfolio with new projects and achievements.
- Remove outdated or less impressive work to maintain quality.
SSL/TLS Certificates Explained: Why Every Website Needs One
What Are SSL/TLS Certificates?
SSL/TLS certificates are digital certificates that authenticate a website’s identity and enable encrypted communication between a web server and a browser. They are essential for ensuring the security and privacy of data transmitted over the internet.
Why Does Every Website Need One?
- Data Encryption: SSL/TLS encrypts data exchanged between the user and the website, protecting sensitive information such as login credentials, payment details, and personal data.
- Authentication: Certificates verify that users are connecting to the legitimate website, not a malicious impostor.
- Trust and Credibility: HTTPS and the padlock icon in the browser address bar boost user confidence.
- SEO Benefits: Search engines like Google prioritize HTTPS websites, improving their search rankings.
- Regulatory Compliance: Many data protection laws and standards, such as GDPR and PCI DSS, require encryption for sensitive data.
Use HTTPS Everywhere
- Always use HTTPS to encrypt data in transit between the client and server.
- Obtain and maintain SSL/TLS certificates from a trusted Certificate Authority (CA).
- Implement HSTS (HTTP Strict Transport Security) headers to force HTTPS connections.
Security Practices for Web Developers
- Validate and Sanitize Input
- Validate all user inputs on both the client and server sides to prevent malicious data.
- Use libraries to sanitize input and remove potentially harmful code, like SQL or HTML scripts.
- Avoid using blacklists; prefer whitelists for acceptable input patterns.
- Implement Strong Authentication
- Enforce strong password policies (e.g., minimum length, complexity).
- Implement multi-factor authentication (MFA) where possible.
- Use secure methods for password storage, such as bcrypt or Argon2, with proper salting and hashing.
- Prevent Cross-Site Scripting (XSS)
- Escape user-generated content before rendering it in HTML.
- Use Content Security Policies (CSP) to restrict the sources of executable scripts.
- Leverage modern frameworks that inherently protect against XSS (e.g., React, Angular).
- Protect Against SQL Injection
- Use parameterized queries or prepared statements to interact with databases.
- Avoid constructing SQL queries by concatenating strings.
- Regularly update database libraries and use ORMs (Object-Relational Mappers) for safer database interaction.
- Secure API Endpoints
- Authenticate and authorize all API requests.
- Implement rate limiting and throttling to prevent abuse.
- Avoid exposing unnecessary information in error messages or API responses.
- Use Secure Cookies
- Mark cookies with
HttpOnly
andSecure
attributes to prevent client-side access. - Use the
SameSite
attribute to protect against Cross-Site Request Forgery (CSRF). - Encrypt sensitive cookie data when possible.
- Mark cookies with
- Handle Sensitive Data Carefully
- Never log or expose sensitive data like passwords, API keys, or tokens.
- Encrypt sensitive data both at rest and in transit.
- Follow data protection regulations, such as GDPR or CCPA, as applicable.
- Monitor and Patch Vulnerabilities
- Regularly update dependencies and frameworks to address known vulnerabilities.
- Use tools like Dependabot, Snyk, or npm audit to monitor for outdated packages.
- Subscribe to security advisories for your tech stack.
- Educate and Train Your Team
- Conduct regular security training and awareness programs for developers.
- Perform code reviews with a focus on security implications.
- Encourage a culture of security-first thinking in your development team.
- Implement Secure Error Handling
- Avoid revealing detailed error messages to users; log them securely instead.
- Use generic error messages to prevent attackers from gaining insights into your system.
- Regular Security Testing
- Conduct regular penetration testing and vulnerability assessments.
- Utilize automated tools for static and dynamic code analysis.
- Simulate attack scenarios, such as phishing or brute force attacks, to evaluate resilience.
- Limit Access and Permissions
- Follow the principle of least privilege (PoLP) for both users and services.
- Use role-based access control (RBAC) to manage permissions effectively.
- Regularly review and audit access permissions.
- Secure Deployment and Configuration
- Store sensitive credentials, such as API keys, in secure environments like secret managers.
- Avoid hardcoding sensitive information in source code.
- Automate security checks in CI/CD pipelines to catch issues early.
By integrating these security practices into the development lifecycle, web developers can significantly reduce the risk of vulnerabilities and build safer, more robust applications.