How to Secure Your Website Against Common Attacks
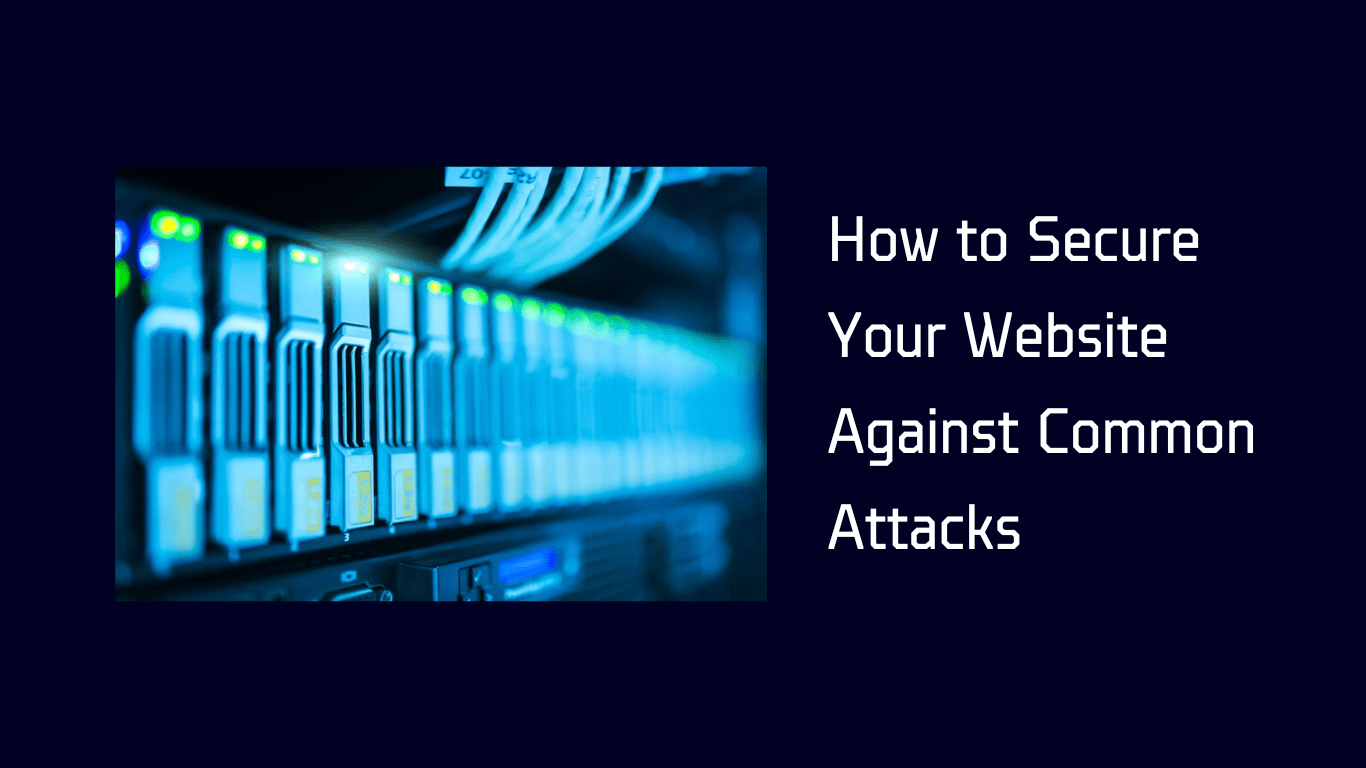
How to Secure Your Website Against Common Attacks
Web development requires not only technical skills but also a deep understanding of security practices to protect applications from potential threats. Here are some essential security practices every web developer should follow:
1. Use HTTPS Everywhere
- Always use HTTPS to encrypt data in transit between the client and server.
- Obtain and maintain SSL/TLS certificates from a trusted Certificate Authority (CA).
- Implement HSTS (HTTP Strict Transport Security) headers to force HTTPS connections.
2. Validate and Sanitize Input
- Validate all user inputs on both the client and server sides to prevent malicious data.
- Use libraries to sanitize input and remove potentially harmful code, like SQL or HTML scripts.
- Avoid using blacklists; prefer whitelists for acceptable input patterns.
3. Implement Strong Authentication
- Enforce strong password policies (e.g., minimum length, complexity).
- Implement multi-factor authentication (MFA) where possible.
- Use secure methods for password storage, such as bcrypt or Argon2, with proper salting and hashing.
4. Prevent Cross-Site Scripting (XSS)
- Escape user-generated content before rendering it in HTML.
- Use Content Security Policies (CSP) to restrict the sources of executable scripts.
- Leverage modern frameworks that inherently protect against XSS (e.g., React, Angular).
5. Protect Against SQL Injection
- Use parameterized queries or prepared statements to interact with databases.
- Avoid constructing SQL queries by concatenating strings.
- Regularly update database libraries and use ORMs (Object-Relational Mappers) for safer database interaction.
6. Secure API Endpoints
- Authenticate and authorize all API requests.
- Implement rate limiting and throttling to prevent abuse.
- Avoid exposing unnecessary information in error messages or API responses.
7. Use Secure Cookies
- Mark cookies with
HttpOnly
andSecure
attributes to prevent client-side access. - Use the
SameSite
attribute to protect against Cross-Site Request Forgery (CSRF). - Encrypt sensitive cookie data when possible.
8. Handle Sensitive Data Carefully
- Never log or expose sensitive data like passwords, API keys, or tokens.
- Encrypt sensitive data both at rest and in transit.
- Follow data protection regulations, such as GDPR or CCPA, as applicable.
9. Monitor and Patch Vulnerabilities
- Regularly update dependencies and frameworks to address known vulnerabilities.
- Use tools like Dependabot, Snyk, or npm audit to monitor for outdated packages.
- Subscribe to security advisories for your tech stack.
10. Educate and Train Your Team
- Conduct regular security training and awareness programs for developers.
- Perform code reviews with a focus on security implications.
- Encourage a culture of security-first thinking in your development team.
11. Implement Secure Error Handling
- Avoid revealing detailed error messages to users; log them securely instead.
- Use generic error messages to prevent attackers from gaining insights into your system.
12. Regular Security Testing
- Conduct regular penetration testing and vulnerability assessments.
- Utilize automated tools for static and dynamic code analysis.
- Simulate attack scenarios, such as phishing or brute force attacks, to evaluate resilience.
13. Limit Access and Permissions
- Follow the principle of least privilege (PoLP) for both users and services.
- Use role-based access control (RBAC) to manage permissions effectively.
- Regularly review and audit access permissions.
14. Secure Deployment and Configuration
- Store sensitive credentials, such as API keys, in secure environments like secret managers.
- Avoid hardcoding sensitive information in source code.
- Automate security checks in CI/CD pipelines to catch issues early.
By integrating these security practices into the development lifecycle, web developers can significantly reduce the risk of vulnerabilities and build safer, more robust applications.