Top Tips for Balancing Front-End and Back-End Workloads as a Full-Stack Developer
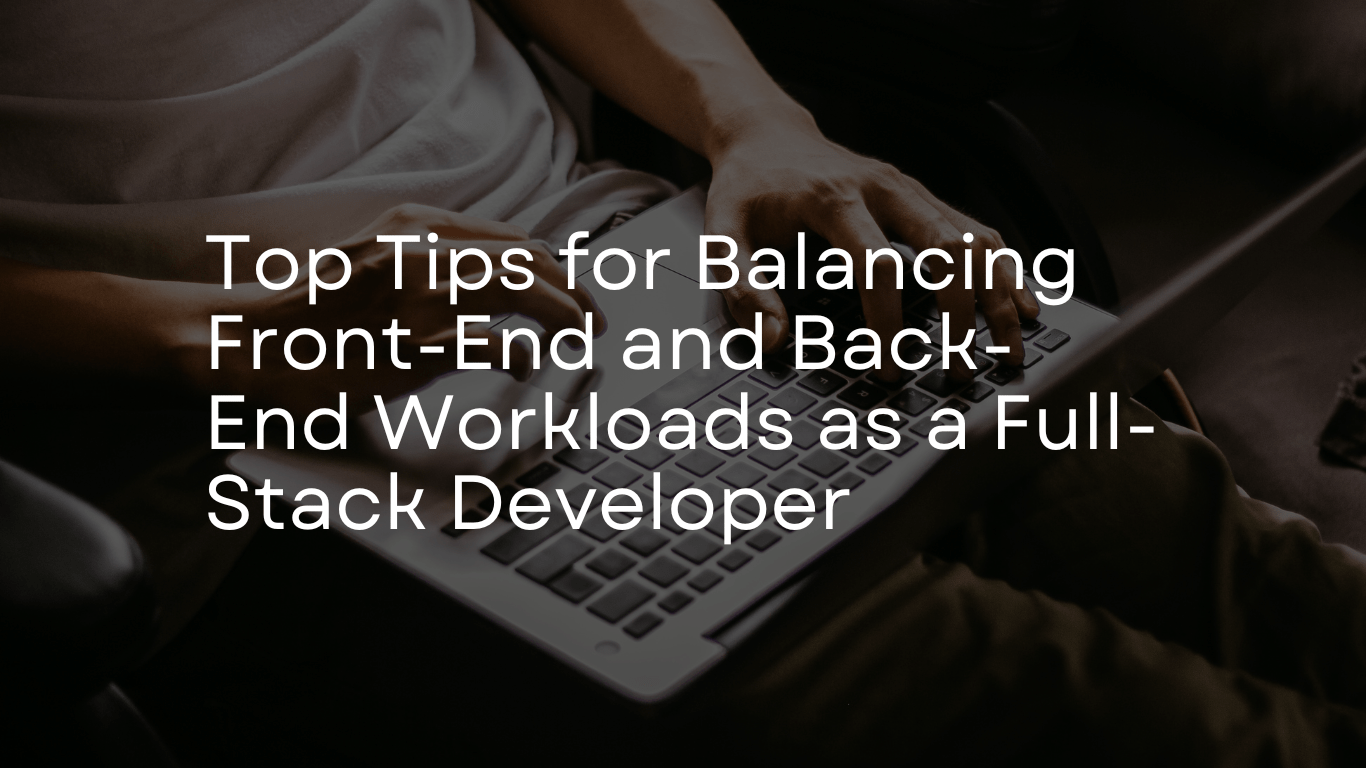
As a full-stack developer, balancing front-end and back-end workloads effectively is crucial for maintaining productivity and delivering high-quality projects. Here are some top tips to help you manage both sides of the stack:
1. Prioritize Communication and Collaboration
- Establish clear expectations: Before starting a project, define the roles and responsibilities for both front-end and back-end tasks. Clear communication with your team (if working in one) will help you set realistic deadlines and priorities.
- Frequent sync-ups: Regularly check in with any team members working on specific parts of the application (e.g., back-end developers or front-end developers) to ensure alignment on goals, data models, and API design.
2. Break Down Tasks and Prioritize
- Chunk tasks: Break down your project into smaller tasks, separating front-end and back-end components. Prioritize which tasks are dependent on others and focus on completing those first. For instance, you may need to set up the back-end API before working on the front-end that consumes it.
- Time-blocking: Allocate distinct time blocks for front-end and back-end work in your schedule. This ensures that you don’t get bogged down in one area while neglecting the other.
3. Master Your Development Environment
- Full-stack development tools: Use integrated development environments (IDEs) or code editors that support both front-end and back-end development, such as Visual Studio Code. Having everything in one place helps with organization and reduces context switching.
- Version control: Use Git (with GitHub, GitLab, etc.) to maintain clear versioning for both the front-end and back-end codebases. This also helps if you are working on multiple parts of the application simultaneously.
- Containerization: Use Docker to create isolated environments for both front-end and back-end. This ensures that both parts of the application run in environments that mimic production, reducing integration issues.
4. Develop Efficient APIs and Communication Layers
- API-first approach: Plan and design your APIs early on. Establish clear routes, request/response formats, and authentication strategies to avoid confusion down the line.
- Mock APIs for front-end development: Use tools like Postman or mock services (e.g., JSONPlaceholder) for simulating back-end responses during front-end development. This allows you to work on the UI even if the back-end isn’t fully implemented yet.
- RESTful or GraphQL APIs: Whether you use RESTful APIs or GraphQL, understanding both will give you flexibility in handling front-end and back-end integration efficiently.
5. Leverage Automation
- Automated testing: Use unit tests and integration tests for both the front-end and back-end to ensure that each layer of your stack functions correctly and that changes in one layer do not break the others.
- For front-end: Use tools like Jest, Mocha, or Cypress for testing components and UI functionality.
- For back-end: Use tools like Jest, Mocha, or Supertest for API testing.
- CI/CD Pipelines: Set up continuous integration and deployment pipelines using services like GitHub Actions, Travis CI, or Jenkins. This automates building, testing, and deploying both front-end and back-end changes, reducing manual overhead.
6. Focus on Modular, Reusable Code
- Component-based architecture: In both front-end and back-end, aim to create reusable modules or components. For example, use React components in the front-end and modularize API routes or services in the back-end.
- Avoid duplication: Maintain clear separation of concerns. Reusing logic across front-end and back-end can reduce redundancy and keep your codebase maintainable.
7. Maintain a Clear Workflow for Deployment
- Unified deployment strategy: Have a clear, synchronized deployment process for both front-end and back-end. Whether you use containerized solutions like Docker, cloud services like AWS, or traditional servers, your front-end and back-end deployments should be tightly coupled.
- Version matching: Ensure that the versions of the front-end and back-end are compatible, particularly if APIs are updated frequently. This helps avoid breaking changes when deploying new updates.
8. Manage Technical Debt
- Refactor regularly: Both front-end and back-end codebases accumulate technical debt over time. Regularly review and refactor your code to prevent any part of the stack from becoming unmanageable.
- Optimize performance: Pay attention to the performance of both your front-end and back-end. For example, optimizing your database queries (back-end) and optimizing the page load times (front-end) will help ensure a smoother user experience.
9. Stay Organized with Task Management Tools
- Use project management tools: Tools like Jira, Trello, or Asana can help you track tasks across the entire stack. Create tasks for both front-end and back-end and monitor progress in one place.
- Track dependencies: Track which tasks are dependent on others (e.g., back-end APIs before front-end integration) to avoid bottlenecks.
10. Learn to Delegate or Use Frameworks
- Know when to ask for help: If you’re a solo developer, know when to delegate front-end or back-end tasks or hire additional help, especially in larger projects. Alternatively, consider using frameworks and libraries (like Next.js or NestJS) that streamline both front-end and back-end development.
- Leverage full-stack frameworks: Some frameworks (like MERN, Django, or Laravel) provide prebuilt components that allow you to focus on the logic rather than the setup of the back-end or front-end, speeding up the development process.
Conclusion
Balancing front-end and back-end work requires planning, organization, and efficient use of tools and techniques. By separating tasks, maintaining clear communication, automating repetitive processes, and staying focused on best practices, you can navigate the demands of full-stack development with ease.